在golang中,make和new都分配内存,但是它们之间仍然存在一些差异。只有了解它们之间的差异,才能在适当的场合使用它们。
简而言之,new只是分配内存,而不初始化内存;make分配并初始化内存。所谓的初始化就是给一个类型赋一个初始值,例如,字符为空,整数为0,逻辑值为false。
从Golang的官方文档的builtin(内置的软件包)中可以找到,make和new的用法。
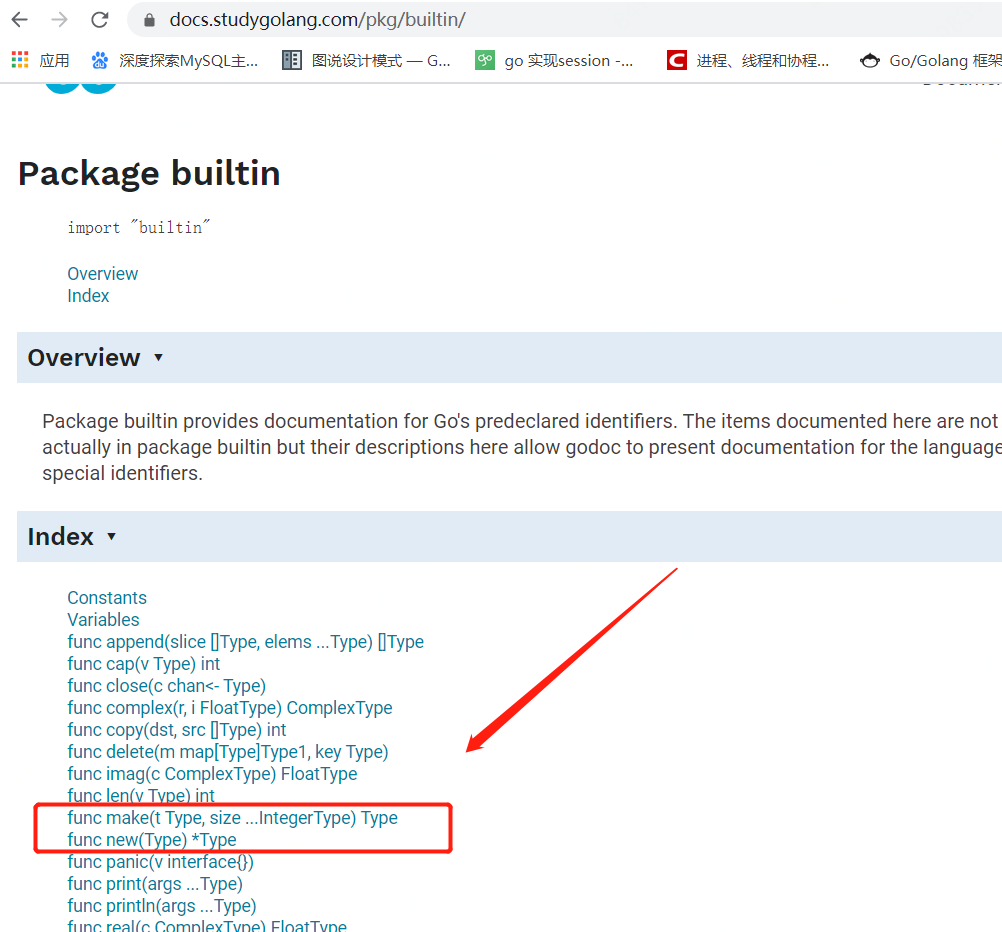
官方文档的内置的软件包
new的使用介绍:
我们先来看看new的定义
- // The new built-in function allocates memory. The first argument is a type,
- // not a value, and the value returned is a pointer to a newly
- // allocated zero value of that type.
- //内建函数new分配内存。其第一个实参为类型,而非值。其返回值为指向该类型的新分配的零值的指针。
- func new(Type) *Type
可以看出,它的参数是一个类型,返回值是指向该类型的内存地址的指针,并且分配的内存将被设置为零,即该类型的零值,即字符为空,整数为0,逻辑值为false
看一些例子
- type P struct {
- Name string
- Age int
- } var a *[2]int
- var s *string
- var b *bool
- var i *int
- var ps *P
- a = new([2]int)
- s = new(string)
- b = new(bool)
- i = new(int)
- ps = new(P) //structure
- fmt.Println(a, " ", *a)
- fmt.Println(s, " ", *s)
- fmt.Println(b, " ", *b)
- fmt.Println(i, " ", *i)
- fmt.Println(ps, " ", *ps)
输出如下:
- &[0 0] [0 0]
- 0xc0000821e0
- 0xc0000a409a false
- 0xc0000a40b0 0
- &{ 0} { 0}
上面基础类型,我们看一下slice, map and channel类型是如何操作的:
- //map 操作
- var mp *map[string]string
- mp = new(map[string]string)
- //注释掉下面的行,new map 返回为nil,直接使用会panic
- //*mp = make(map[string]string) // if this line is omitted, it will pan "Pan: assignment to entry in nil map"“
- (*mp)["name"] = "lc"
- fmt.Println((*mp)["name"])
- // slice 操作 var ms *[]string
- ms = new([]string)
- // 注释掉下面的行访问的时候会下标超出范围 //*ms = make([]string,5) // if this line is deleted, it will "panic: runtime error: index out of range"
- (*ms)[0] = "lc"
- fmt.Println((*ms)[0])
从上面可以看出,silce,map,channel和其他类型是引用类型。当引用类型初始化为nil时,不能直接分配nil,也不能使用new来分配内存,还需要使用make来进行分配。
make的使用介绍:
我们看一下make的定义
- / /The make built-in function allocates and initializes an object of type
- // slice, map, or chan (only). Like new, the first argument is a type, not a
- // value. Unlike new, make's return type is the same as the type of its
- // argument, not a pointer to it. The specification of the result depends on
- // the type:
- // Slice: The size specifies the length. The capacity of the slice is
- // equal to its length. A second integer argument may be provided to
- // specify a different capacity; it must be no smaller than the
- // length. For example, make([]int, 0, 10) allocates an underlying array
- // of size 10 and returns a slice of length 0 and capacity 10 that is
- // backed by this underlying array.
- // Map: An empty map is allocated with enough space to hold the
- // specified number of elements. The size may be omitted, in which case
- // a small starting size is allocated.
- // Channel: The channel's buffer is initialized with the specified
- // buffer capacity. If zero, or the size is omitted, the channel is
- // unbuffered.
- //切片:size指定了其长度。该切片的容量等于其长度。切片支持第二个整数实参可用来指定不同的容量; 它必须不小于其长度,因此 make([]int, 0, 10) 会分配一个长度为0,容量为10的切片。
- //映射:初始分配的创建取决于size,但产生的映射长度为0。size可以省略,这种情况下就会分配一个小的起始大小。
- //通道:通道的缓存根据指定的缓存容量初始化。若 size为零或被省略,该信道即为无缓存的。
- func make(t Type, size ...IntegerType) Type
可以看出,它返回的是类型本身而不是指针类型,因为make只能为slice,map,channel等初始化内存,并且它们返回引用类型,因此不必返回指针
让我们看一些make的例子:
- mm :=make(map[string]string)
- mm["name"] = "lc"
- fmt.Println(mm["name"])
- mss :=make([]int,2)
- mss[0] = 100
- fmt.Println(mss[0])
- ch :=make(chan int,1)
- ch <-100
- fmt.Println(<-ch)
总结:
make仅用于分配和初始化slice,map和chan类型的数据。new可以分配任何类型的数据。new分配返回一个指针,即Type * Type。make返回一个引用,该引用为Type由make分配的空间之后,清除并初始化由new分配的空间。